« Go back
Texture your Shapes
Do you remember what Shapes were good for? As the name suggests, you can nicely draw primitive Shapes with them. But you can do more. Shapes are also a nice possibility to bind Textures to.
This time we will therefore create a Shape and then bind a Texture to it:
Show Raw
import std.stdio; import Dgame.Window.Window; import Dgame.Window.Event; import Dgame.Graphic; import Dgame.Math.Geometry; import Dgame.Math.Vertex; void main() { Window wnd = Window(640, 480, "Dgame Test"); Surface wiki_srfc = Surface("samples/images/wiki.png"); Texture wiki_tex = Texture(wiki_srfc); Shape texQuad = new Shape( Geometry.Quad, [ Vertex( 0, 0), Vertex(140, 0), Vertex(140, 140), Vertex( 0, 140) ] ); texQuad.setTexture(&wiki_tex); texQuad.setColor(Color4b.Green.withTransparency(125)); texQuad.setPosition(200, 100); texQuad.setRotation(25); bool running = true; Event event; while (running) { wnd.clear(); while (wnd.poll(&event)) { switch (event.type) { case Event.Type.Quit: writeln("Quit Event"); running = false; break; default: break; } } wnd.draw(texQuad); wnd.display(); } }
You should see something like this:
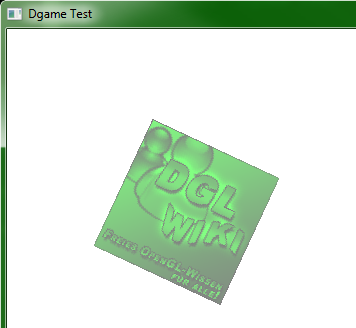
Of course you can change the Geometry to e.g. Geometry.Triangle
to see the image blitted on a triangle Shape like this:
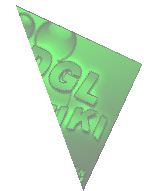
For me, the square looks quite lonely. Let's give him at last company in the form of a rotated, textured circle:
Show Raw
import std.stdio; import Dgame.Window.Window; import Dgame.Window.Event; import Dgame.Graphic; import Dgame.Math; void main() { Window wnd = Window(640, 480, "Dgame Test"); Surface wiki_srfc = Surface("samples/images/wiki.png"); Texture wiki_tex = Texture(wiki_srfc); Shape texQuad = new Shape( Geometry.Quad, [ Vertex( 0, 0), Vertex(140, 0), Vertex(140, 140), Vertex( 0, 140) ] ); texQuad.setTexture(&wiki_tex); texQuad.setColor(Color4b.Green.withTransparency(125)); texQuad.setPosition(200, 100); texQuad.setRotation(25); Shape texCircle = new Shape(50, Vector2f(50, 50)); texCircle.setTexture(&wiki_tex); texCircle.setTextureRect(Rect(25, 25, 100, 100)); texCircle.setColor(Color4b.White); texCircle.move(400, 300); texCircle.setRotation(25); bool running = true; Event event; while (running) { wnd.clear(); while (wnd.poll(&event)) { switch (event.type) { case Event.Type.Quit: writeln("Quit Event"); running = false; break; default: break; } } wnd.draw(texQuad); wnd.draw(texCircle); wnd.display(); } }
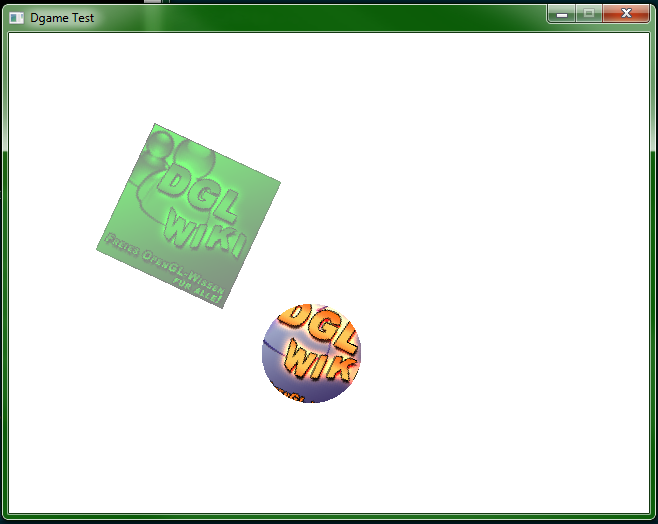
« Go back