« Go back
OpenGL Settings : Anti Aliasing
As you may have noticed, if you draw Shapes (especially circles) the edges are not smooth. That's because anti aliasing is disabled as default option because anti aliasing is a very costly option which draws nice and smooth lines but decreases the framerate. But nevertheless, if you want to use it, here is how to do it:
import std.stdio; import Dgame.Window; import Dgame.Graphic; import Dgame.Math; import Dgame.System; void main() { GLContextSettings gl_settings; gl_settings.antiAlias = GLContextSettings.AntiAlias.X4; gl_settings.vers = GLContextSettings.Version.GL30; Window wnd = Window(640, 480, "Dgame Test", Window.Style.Default, gl_settings); wnd.setVerticalSync(Window.VerticalSync.Enable); wnd.setClearColor(Color4b.Gray); Shape qs = new Shape(Geometry.Quads, [ Vertex( 75, 75), Vertex(275, 75), Vertex(275, 275), Vertex( 75, 275) ] ); qs.fill = Shape.Fill.Full; qs.setColor(Color4b.Blue); qs.setPosition(200, 50); Shape circle = new Shape(25, Vector2f(180, 380)); circle.setColor(Color4b.Green); circle.setPosition(150, -100); bool running = true; Event event; while (running) { wnd.clear(); while (wnd.poll(&event)) { switch (event.type) { case Event.Type.Quit: writeln("Quit Event"); running = false; break; case Event.Type.KeyDown: if (event.keyboard.key == Keyboard.Key.T) { if (qs.geometry == Geometry.Quads) qs.geometry = Geometry.Triangles; else qs.geometry = Geometry.Quads; } else if (event.keyboard.key == Keyboard.Key.Space) circle.move(4, -4); break; default: break; } } wnd.draw(qs); wnd.draw(circle); wnd.display(); } }
That should result in something like this:
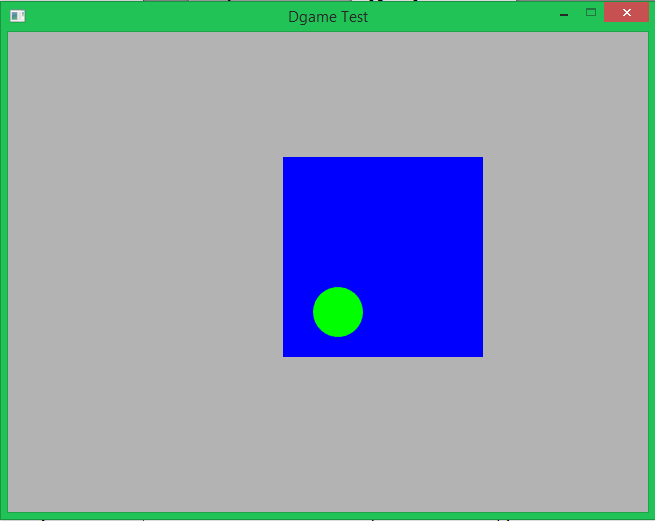
You may note the the GLContextSettings struct here
GLContextSettings gl_settings; gl_settings.antiAlias = GLContextSettings.AntiAlias.X4; gl_settings.vers = GLContextSettings.Version.GL30;
which is used with vers
and antiAlias
.
vers
is the major and minor version of OpenGL as an enum member. This enum holds all valid values for OpenGL versions you can use. antiAlias
is the anti-aliasing level. AntiAlias
is also an enum which holds all valid values for the anti-aliasing level. Higher levels result in nicer smooth lines, but you should be aware that this is a very expensive operation that reduces the frame rate drastically.
Comparison
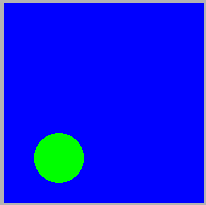
Anti-aliasing level of 0 (AntiAlias.None
)
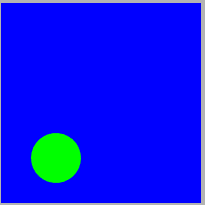
Anti-aliasing level of 8 (AntiAlias.X8
)
« Go back